PHP is a comprehensive and powerful language that has seen a meteoric rise over the past decade. I started working with PHP back in 1998 when I ran a gaming community on Crossroads Gaming, now known as the Warcry Network. I did a lot of what I’m doing now… connecting to databases, pulling information based on given criteria and then presenting it on a page in an attractive and organized manner.
These days, things have changed a little bit… we’re using templating to display information and everything is object oriented. We’re dealing with automatically loaded classes and closures and, for all intents and purposes, it gets what we need done in the realm of the world wide web. But there’s another aspect of PHP that is, in my opinion, underutilized: command line scripting.
The obvious usefulness to a web developer may not be readily apparent, but when you need to combine systems programming to maintain elements of site maintenance or for outright data processing jobs, using the command line interface is a great option. Let me give you a ‘For Instance…”
For instance, here at Delaware Tech, I wrote a web-based tool that allows the administration to send out thousands of emails to students in the event of an emergency (weather related closings, threat on campus, etc). If the process of sending these emails out were based on the function of the web page, there would be drastic repercussions if the person’s computer crashed or the browser locked up in the middle of generating the emails.
Instead, the webpage simply writes a file with a list of email addresses and the ‘to’, ‘from’, subject and body of the email. Every minute, the cron process fires off a PHP script that checks for the file and runs the process on the server, independent of any web-based session.
If you have a site that requires hourly or daily functions or you want to batch process a CSV file, then read on… it’s simpler than you think!
Here’s a super simple PHP CLI script to email select users based on information from a comma separated value file:
#!/usr/bin/php //This has your database password and a simple MySQL connection. //Use it to establish a connection. include('database_info.php'); mysqli_connect($dbhost, $dbuser, $dbpass); mysqli_select($dbname); //This is a comma separated value file in the following format: //userID,FName,LName -- for example: //1,Rob,Wiltbank //6,Fred,Flintstone $fp = fopen("UsersToEmail.csv", "r"); //Go through the file line by line. while(($buffer = fgets($handle, 4096)) !== false) { //Explode the line, so $user[0] will be the userID,</span> //$user[1] will be FName and $user[2] will be LName. $user = explode(',', $buffer); //Assuming we know the list contains all valid users and //there's only a single row per user. $sql = "SELECT email FROM user_table WHERE userID = {$user[0]}"; $result = mysql_query($sql); $row = mysql_fetch_assoc($result); //Now email the user. mail($row['email'], 'Confirmation Email', "Here's your confirmation, {$user[1]} {$user[2]}!"); }
So, in reality, what do I really use this for at the college?
Well, I have 7 cron jobs that pull student information, format them into a CSV and upload them to a contracted vendor for scholarship management, educational material enrollment or other value-added services. I have 3 crons that check for new students who have identified themselves as veterans and send appropriate confirmation emails to the student and to their Counselor to verify their status. I have 1 cron that takes tutoring survey information submitted that day and sends a summary to the instructors. I have a cron that also prunes the password tokens we create for online admissions daily. And, as previously mentioned there are several jobs that check for general emergency and mass notification jobs pending to send those emails out.
On top of this, I have several data scrubbing and parsing scripts that let me take files and pull missing information (if I need emails, usernames, etc), ones that allow me to pull reports and see how many students still need our newly formatted ID badges and one that lets me verify no active student or staff accounts will get deleted during our quarterly purge.
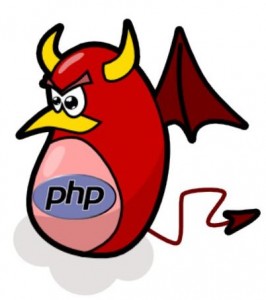
PHP Daemon
What’s the extent of this?
In all honesty, the sky is the limit. For quite some time now, PHP has had built-in support for sockets allowing you to create PHP-based daemon processes. That is, scripts that always run and will listen on a given network port for a command.
This can be leveraged into building your own Instant Messenger protocol, an socket-based API-driven framework or even an online game server!
But, that is another post. 🙂
In the meantime, if you stumble across a need to process some information, cross-reference a database or handle some batch task, look to PHP. It will serve you well.
Leave a Comment
You must be logged in to post a comment.